PIL图像转换为Tensor
在Pytorch中,PIL图像可以通过以下方式转换为Tensor:
import torch
from PIL import Image
image = Image.open('your_image.png')
tensor_img = torch.from_numpy(np.array(image)).permute(2, 0, 1).float()/255.0
print(tensor_img)
print(tensor_img.shape)
其中,np.array()将PIL Image转换为numpy数组,.permute()调整了数组的维度以适应Pytorch Tensor的格式,并将数据类型转换为float类型。
tensor([[[0.5137, 0.5137, 0.5216, ..., 1.0000, 1.0000, 1.0000],
[0.5098, 0.5137, 0.5176, ..., 1.0000, 1.0000, 1.0000],
[0.5020, 0.5020, 0.5098, ..., 1.0000, 1.0000, 1.0000],
...,
[0.2314, 0.2314, 0.2431, ..., 0.2157, 0.2157, 0.2392],
[0.2314, 0.2275, 0.2353, ..., 0.2157, 0.2196, 0.2510],
[0.2627, 0.2588, 0.2510, ..., 0.2157, 0.2118, 0.2392]],
[[0.4824, 0.4824, 0.4902, ..., 1.0000, 1.0000, 1.0000],
[0.4784, 0.4824, 0.4863, ..., 1.0000, 1.0000, 1.0000],
[0.4706, 0.4706, 0.4784, ..., 1.0000, 1.0000, 1.0000],
...,
[0.3137, 0.3059, 0.3176, ..., 0.3059, 0.3059, 0.3294],
[0.3137, 0.3020, 0.3098, ..., 0.3059, 0.3098, 0.3412],
[0.3373, 0.3412, 0.3333, ..., 0.3059, 0.3020, 0.3294]],
[[0.4706, 0.4706, 0.4784, ..., 1.0000, 1.0000, 1.0000],
[0.4667, 0.4706, 0.4745, ..., 1.0000, 1.0000, 1.0000],
[0.4588, 0.4588, 0.4667, ..., 1.0000, 1.0000, 1.0000],
...,
[0.4196, 0.4235, 0.4353, ..., 0.4275, 0.4275, 0.4510],
[0.4196, 0.4196, 0.4275, ..., 0.4275, 0.4314, 0.4627],
[0.4549, 0.4471, 0.4392, ..., 0.4275, 0.4235, 0.4510]]])
Tensor转换为PIL图像
将Tensor转换为PIL图像可以使用以下代码:
import numpy as np
from PIL import Image
tensor = tensor_img
tensor = tensor.cpu().clone()
tensor = tensor.squeeze(0)
tensor = tensor.permute(1, 2, 0)
image = tensor.numpy()
image = (image * 255).astype(np.uint8)
image = Image.fromarray(image)
首先,将Tensor复制到CPU并调整维度。然后使用.numpy()函数将Tensor转换为numpy数组,并乘以255以还原为原始图像数据类型。最后使用Image.fromarray()将numpy数组转换为PIL Image。
输出为:
(4000, 2250, 3)
[[[131 123 120]
[131 123 120]
[133 125 122]
...
[255 255 255]
[255 255 255]
[255 255 255]]
[[130 122 119]
[131 123 120]
[132 124 121]
...
[255 255 255]
[255 255 255]
[255 255 255]]
[[128 120 117]
[128 120 117]
[130 122 119]
...
[255 255 255]
[255 255 255]
[255 255 255]]
...
...
[ 55 78 109]
[ 54 77 108]
[ 61 84 115]]]
验证 PIL 再次转为 tensor
import torch
from PIL import Image
tensor_img = torch.from_numpy(np.array(image)).permute(2, 0, 1).float()/255.0
print(tensor_img)
print(tensor_img.shape)
输出为
tensor([[[0.5137, 0.5137, 0.5216, ..., 1.0000, 1.0000, 1.0000],
[0.5098, 0.5137, 0.5176, ..., 1.0000, 1.0000, 1.0000],
[0.5020, 0.5020, 0.5098, ..., 1.0000, 1.0000, 1.0000],
...,
[0.2314, 0.2314, 0.2431, ..., 0.2157, 0.2157, 0.2392],
[0.2314, 0.2275, 0.2353, ..., 0.2157, 0.2196, 0.2510],
[0.2627, 0.2588, 0.2510, ..., 0.2157, 0.2118, 0.2392]],
[[0.4824, 0.4824, 0.4902, ..., 1.0000, 1.0000, 1.0000],
[0.4784, 0.4824, 0.4863, ..., 1.0000, 1.0000, 1.0000],
[0.4706, 0.4706, 0.4784, ..., 1.0000, 1.0000, 1.0000],
...,
[0.3137, 0.3059, 0.3176, ..., 0.3059, 0.3059, 0.3294],
[0.3137, 0.3020, 0.3098, ..., 0.3059, 0.3098, 0.3412],
[0.3373, 0.3412, 0.3333, ..., 0.3059, 0.3020, 0.3294]],
[[0.4706, 0.4706, 0.4784, ..., 1.0000, 1.0000, 1.0000],
[0.4667, 0.4706, 0.4745, ..., 1.0000, 1.0000, 1.0000],
[0.4588, 0.4588, 0.4667, ..., 1.0000, 1.0000, 1.0000],
...,
[0.4196, 0.4235, 0.4353, ..., 0.4275, 0.4275, 0.4510],
[0.4196, 0.4196, 0.4275, ..., 0.4275, 0.4314, 0.4627],
[0.4549, 0.4471, 0.4392, ..., 0.4275, 0.4235, 0.4510]]])
正文完
可以使用微信扫码关注公众号(ID:xzluomor)
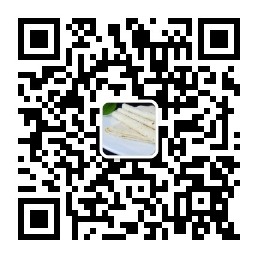